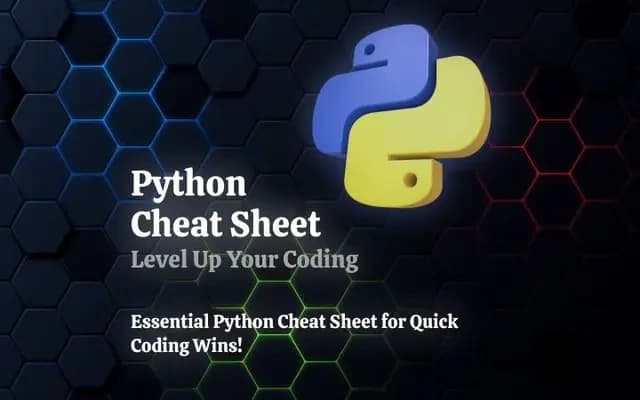
Python Cheat-Sheet: Your Quick Reference Guide
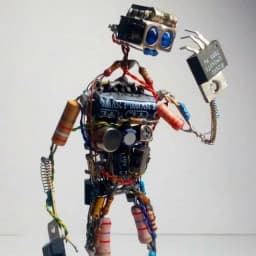
Published on 23rd Jul, 2023
25 min read
Welcome to our comprehensive Python Cheat Sheet, designed to be your go-to quick reference guide for mastering Python programming. Whether you're a seasoned developer or just starting your coding journey, having a cheat sheet at your fingertips can significantly boost your productivity and efficiency.
Python is a versatile and powerful programming language known for its readability and ease of use. It's widely used in web development, data analysis, artificial intelligence, automation, and many other domains. With Python's extensive libraries and straightforward syntax, learning and using Python has never been more accessible.
In this blog, we have compiled a concise yet comprehensive Python Cheat Sheet, covering essential concepts, functions, and tips that every Python developer should know. From basic data types and control flow to advanced topics like object-oriented programming and file handling, this cheat sheet has you covered.
Whether you need a quick reminder of Python's syntax, a refresher on how to use lists and dictionaries effectively, or guidance on handling exceptions, our cheat sheet has it all. We've also included essential Python libraries and their usage to help you harness the power of Python's ecosystem.
No more searching through lengthy documentation or tutorials when you're stuck on a coding problem. With this cheat sheet in hand, you can quickly find the information you need and get back to writing efficient and clean Python code.
Mastering Basic Syntaxes
Welcome to the Python Cheat Sheet dedicated to mastering the fundamental syntax of Python programming. Understanding the basic syntax is the first step towards becoming proficient in Python. In this section, we will cover essential Python syntax elements, from variables and data types to control structures and functions. Whether you're a beginner or need a quick refresher, this cheat sheet will serve as your invaluable companion in navigating the world of Python programming. Let's dive in and uncover the building blocks of this versatile and powerful language!
Python Syntax compared to other programming languages
- Python was designed for readability, and has some similarities to the English language with influence from mathematics.
- Python uses new lines to complete a command, as opposed to other programming languages which often use semicolons or parentheses.
- Python relies on indentation, using whitespace, to define scope; such as the scope of loops, functions and classes. Other programming languages often use curly brackets for this purpose.
Variables
Variables are containers for storing data values. Unlike other programming languages, Python has no command for declaring a variable. A variable is created the moment you first assign a value to it.
Variables do not need to be declared with any particular type and can even change type after they have been set.
Python allows you to assign values to multiple variables in one line.
x = 5 # Integer
y = True # Boolean
z = "Hello, World!" # String
z = 'Hello World' # String can be single quoted
z = """Multiline
String"""
x = "Arif Sardar" # Can re-assign values to a variable even the data-type
x, y, z = "Orange", "Banana", "Cherry" # Assign multiple variables in one line
x = y = z = "Orange" # Assign same value to multiple variables
Output Variables
The Python print statement is often used to output variables.
print("Python is awesome!")
x = 'Python'
print(x + ' is awesome') # Can use all operators or call functions in print function
print(23 + 47) # Output: 70
Global Variables
Variables that are created outside of a function (as in all of the examples above) are known as global variables. Global variables can be used by everyone, both inside of functions and outside.
x = "awesome" # Global variable, can be used everywhere
def myfunc():
print("Python is " + x)
myfunc()
The global Keyword
Normally, when you create a variable inside a function, that variable is local, and can only be used inside that function. To create a global variable inside a function, you can use the global keyword.
def myfunc():
global x # declare the variable as global
x = "fantastic" # Assign value to it
myfunc()
print("Python is " + x) # Output: Python is fantastic
Comments
Python has commenting capability for byte arrayin-code documentation. Comments start with a #, and Python will render the rest of the line as a comment:
# This is a comment.
print("Hello, World!")
'''
This is a
multiline
comment
'''
Built-in Data Types
In programming, data type is an important concept. Variables can store data of different types, and different types can do different things.
Python has the following data types built-in by default, in these categories:
Types | Examples |
---|---|
Text | str |
Numeric | int, float, complex |
Sequence | list, tuple, range |
Mapping | dict |
Set | set, frozenset |
Boolean | bool |
Binary | bytes, bytearray, memoryview |
Getting the Data Type
You can get the data type of any object by using the type() function:
x = 5
print(type(x)) # Output: int
Here arethe some example datatypes and their uses:
Example Data | Type |
---|---|
x = "Hello World" | str |
x = 20 | int |
x = 20.5 | float |
x = 1j | complex |
x = ["apple", "banana", "cherry"] | list |
x = ("apple", "banana", "cherry") | tuple |
x = range(6) | range |
x = {"name": "John", “age”: 36} | dict |
x = {"apple", "banana", "cherry"} | set |
x = frozenset({"apple", "banana", "cherry"}) | frozenset |
x = True | bool |
x = b"Hello" | bytes |
x = bytearray(5) | bytearray |
x = memoryview(bytes(5)) | memoryview |
Setting the Data Type
In Python, the data type is set when you assign a value to a variable:
Setting the Specific Data Type
If you want to specify the data type, you can use the following constructor functions:
x = str("Hello World") # str
x = int(20) # int
x = float(20.5) # float
x = complex(1j) # complex
x = list(("apple", "banana", "cherry")) # list
x = tuple(("apple", "banana", "cherry")) # tuple
x = range(6) # range
x = dict(name="John", age=36) # dict
x = set(("apple", "banana", "cherry")) # set
x = frozenset({"apple", "banana", "cherry"}) # frozenset
x = bool(5) # bool
x = bytes(5) # bytes
x = bytearray(5) # bytearray
x = memoryview(bytes(5)) # memoryview
Type Conversion
You can convert from one type to another with the int(), float(), and complex() methods:
x = 1 # int
y = 2.8 # float
z = 1j # complex
#convert from int to float:
a = float(x)
#convert from float to int:
b = int(y)
#convert from int to complex:
c = complex(x)
print(a) # 1.0
print(b) # 2
print(c) # (1+0j)
print(type(a)) # <class 'float'>
print(type(b)) # <class 'int'>
print(type(c)) # <class 'complex'>
Python Operators
Operators are used to perform operations on variables and values. Python divides the operators into the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Identity operators
- Membership operators
- Bitwise operators
Python Arithmetic Operators
Arithmetic operators are used with numeric values to perform common mathematical operations:
Operator | Name | Example |
---|---|---|
+ | Addition | x + y |
- | Subtraction | x - y |
* | Multiplication | x * y |
/ | Division | x / y |
% | Modulus | x % y |
** | Exponentiation | x ** y OR, x ^ y |
// | Floor division | x // y |
Python Assignment Operators
Assignment operators are used to assign values to variables:
Operator | Example | Same As |
---|---|---|
= | x = 5 | x = 5 |
+= | x += 3 | x = x + 3 |
-= | x -= 3 | x = x - 3 |
*= | x *= 3 | x = x * 3 |
/= | x /= 3 | x = x / 3 |
%= | x %= 3 | x = x % 3 |
//= | x //= 3 | x = x // 3 |
**= | x **= 3 | x = x ** 3 |
&= | x &= 3 | x = x & 3 |
|= | x |= 3 | x = x | 3 |
^= | x ^= 3 | x = x ^ 3 |
>>= | x >>= 3 | x = x >> 3 |
<<= x | <<= 3 | x = x << 3 |
Python Comparison Operators
Comparison operators are used to compare two values:
Operator | Name | Example |
---|---|---|
== | Equal | x == y |
!= | Not equal | x != y |
> | Greater than | x > y |
< | Less than | x < y |
>= | Greater than or equal to | x >= y |
<= | Less than or equal to | x <= y |
Python Logical Operators
Logical operators are used to combine conditional statements:
Operator | Description | Example |
---|---|---|
and | Returns True if both statements are true | x < 5 and x < 10 |
or | Returns True if one of the statements is true | x < 5 or x < 4 |
not | Reverse the result, returns False if the result is true | not(x < 5 and x < 10) |
Python Identity Operators
Identity operators are used to compare the objects, not if they are equal, but if they are actually the same object, with the same memory location:
Operator | Description | Example |
---|---|---|
is | Returns True if both variables are the same object | x is y |
is not | Returns True if both variables are not the same object | x is not y |
Python Membership Operators
Membership operators are used to test if a sequence is presented in an object:
Operator | Description | Example |
---|---|---|
in | Returns True if a sequence with the specified value is present in the object | x in y |
not in | Returns True if a sequence with the specified value is not present in the object | x not in y |
Python Bitwise Operators
Bitwise operators are used to compare (binary) numbers:
Operator | Name | Description |
---|---|---|
& | AND | Sets each bit to 1 if both bits are 1 |
| | OR | Sets each bit to 1 if one of two bits is 1 |
^ | XOR | Sets each bit to 1 if only one of two bits is 1 |
~ | NOT | Inverts all the bits |
<< | Zero-fill left shift | Shift left by pushing zeros in from the right and let the leftmost bits fall off |
>> | Signed right shift | Shift right by pushing copies of the leftmost bit in from the left, and let the rightmost bits fall off |
Strings
We can define strings using single (‘ ‘) or double (“ “) quotes. To define a multi-line string, we surround our string with tripe quotes (“””).
z = "Hello, World!" # String
z = 'Hello World' # String can be single quoted
z = """Multiline
String"""
We can get individual characters in a string using square brackets []. We can slice a string using a similar notation.
course = "Python for Beginners"
course[0] # returns the first character (P)
course[1] # returns the second character (y)
course[-1] # returns the first character from the end (s)
course[-2] # returns the second character from the end (r)
course[1:5] # Output: ytho
# returns the characters from index position 1 to 5 but excluding 5.
# If we leave out the start index, 0 will be assumed.
# If we leave out the end index, the length of the string will be assumed.
We can use formatted strings to insert values into our strings dynamically:
name = "Arif"
message = f"Hi, my name is {name}"
String Methods
x = "Hello, World!"
x.title() # To capitalize the first letter of every word
x.find("p") # Returns the index of the first occurrence of p (or -1 if not found)
x.replace("p", "q") # Replaces all 'p' with 'q'
x.isalnum() # Returns True if all the characters in the string are alphanumeric, else False
x.isalpha() # Returns True if all the characters in the string are alphabets
x.isdecimal() # Returns True if all the characters in the string are decimals
x.isdigit() # Returns True if all the characters in the string are digits
x.islower() # Returns True if all characters in the string are lower case
x.isspace() # Returns True if all characters in the string are whitespaces
x.isupper() # Returns True if all characters in the string are upper case
x.lower() # Converts a string into lower case equivalent
x.upper() # Converts a string into upper case equivalent
x.strip() # It removes leading and trailing spaces in the string
To check if a string contains a character (or a sequence of characters), we use the in operator:
contains = "Python" in course
Escape Sequence
An escape sequence is a sequence of characters; it doesn't represent itself (but is translated into another character) when used inside a string literal or character. Some of the escape sequence characters are as follows:
print("\n") # New Line
print("\\") # Backslash
print("\'") # Single Quote
print("\t") # Tab Space
print("\b") # Backspace
print("\ooo") # Octal Value
print("\xhh") # Hex Value
print("\r") # Carriage Return (Carriage return or \r will just work as you have shifted your cursor to the beginning of the string or line.)
Python Collections (Arrays)
There are four collection data types in the Python programming language:
**List**
is a collection that is ordered and changeable. Allows duplicate members.**Tuple**
is a collection that is ordered and unchangeable. Allows duplicate members.**Set**
is a collection that is unordered and unindexed. No duplicate members.**Dictionary**
is a collection that is unordered, changeable and indexed. No duplicate members.
1. List
A List in Python represents a list of comma-separated values of any data type between square brackets.
var_name = [element1, element2, ...]
my_list = [] # Empty List
Note: These elements can be of different datatypes.
Indexing: The position of every element placed in the string starts from the 0th position and step by step it ends at length-1 position.
The list is ordered, indexed, mutable and the most flexible and dynamic collection of elements in Python.
List Methods
list.index(element) # Returns the index of the first element with the specified value
list.append(element) # Adds an element at the end of the list
list.extend(iterable) # Add the elements of a given list (or any iterable) to the end of the current list
list.insert(position, element) # Adds an element at the specified position
list.pop(position) # Removes the element at the specified position and returns it
list.remove(element) # The remove() method removes the first occurrence of a given item from the list
list.clear() # Removes all the elements from the list
list.count(value) # Returns the number of elements with the specified value
list.reverse() # Reverses the order of the list
list.sort(reverse=True|False) # Sorts the list
2. Tuples
Tuples are represented as comma-separated values of any data type within parentheses.
variable_name = (element1, element2, ...)
Note: These elements can be of different datatypes
Indexing: The position of every element placed in the string starts from the 0th position and step by step it ends at length-1 position
Tuples are ordered, indexing, immutable and the most secured collection of elements.
Tuple Methodes
tuple.count(value) # It returns the number of times a specified value occurs in a tuple
tuple.index(value) # It searches the tuple for a specified value and returns the position.
3. Sets
A set is a collection of multiple values which is both unordered and unindexed. It is written in curly brackets.
var_name = {element1, element2, ...}
var_name = set([element1, element2, ...])
Set is an unordered, immutable,non-indexed type of collection. Duplicate elements are not allowed in sets.
Set Methods
set.add(element) # Adds an element to a set
set.clear() # Remove all elements from a set
set.discard(value) # Removes the specified item from the set
set.intersection(set1, set2 ... etc) # Returns intersection of two or more sets
set.issubset(set) # Checks if a set is a subset of another set
set.pop() # Removes an element from the set
set.remove(item) # Removes the specified element from the set
set.union(set1, set2...) # Returns the union of two or more sets
4. Dictionaries
The dictionary is an unordered set of comma-separated key: value pairs, within , with the requirement that within a dictionary, no two keys can be the same.
<dictionary-name> = {<key>: value, <key>: value ...}
mydict={} # Empty Dictionary
<dictionary>[<key>] = <value> # Adding Element to a dictionary
<dictionary>[<key>] = <value> # Updating Element in a dictionary
del <dictionary>[<key>] # Deleting an element from a dictionary
A dictionary is an ordered and mutable collection of elements. The dictionary allows duplicate values but not duplicate keys.
Dictionary Methods
len(dictionary) # It returns the length of the dictionary, i.e., the count of elements (key: value pairs) in the dictionary
dictionary.clear() # Removes all the elements from the dictionary
dictionary.get(keyname) # Returns the value of the specified key
dictionary.items() # Returns a list containing a tuple for each key-value pair
dictionary.keys() # Returns a list containing the dictionary's keys
dictionary.values() # Returns a list of all the values in the dictionary
dictionary.update(iterable) # Updates the dictionary with the specified key-value pairs
Python Conditions
The if, elif and else statements are the conditional statements in Python, and these implement selection constructs (decision constructs).
1. if Statement
if(conditional expression):
statements
2. if-else Statement
if(conditional expression):
statements
else:
statements
3. if-elif Statement
if (conditional expression):
statements
elif (conditional expression):
statements
else:
statements
4. Nested if-else Statement
if (conditional expression):
if (conditional expression):
statements
else:
statements
else:
statements
Python Loops
A loop or iteration statement repeatedly executes a statement, known as the loop body, until the controlling expression is false (0). Python has two primitive loop commands:
1. For Loop
The for loop of Python is designed to process the items of any sequence, such as a list or a string, one by one.
This is less like the keyword in other programming languages and works more like an iterator method as found in other object-orientated programming languages.
for <variable> in <sequence>:
statements_to_repeat
2. While Loop
A while loop is a conditional loop that will repeat the instructions within itself as long as a conditional remains true.
while <logical-expression>:
loop-body
Break Statement
The break statement enables a program to skip over a part of the code. A break statement terminates the very loop it lies within.
for <var> in <sequence>:
statement1
if <condition>:
break
statement2
statement_after_loop
Continue Statement
The continue statement skips the rest of the loop statements and causes the next iteration to occur.
for <var> in <sequence>:
statement1
if <condition>:
continue
statement2
statement3
statement4
Functions
A function is a block of code that performs a specific task when it is called. You can pass parameters into a function. It helps us to make our code more organized and manageable. A function can return data as a result.
Function Definition:
The “def” keyword is used before defining the function.
def my_function():
print("Hello from a function")
Function Call:
def my_function(): # Function Definition
print("Hello from a function")
my_function() # Function Call
Parameters / Arguments:
Whenever we need that block of code in our program simply call that function name whenever needed. If parameters are passed during defying the function we have to pass the parameters while calling that function. example-
def my_function(fname): # Function Definition
print(fname + " Refsnes")
my_function("Emil") # OUTPUT: Emil Refsnes
my_function("Tobias") # OUTPUT: Tobias Refsnes
my_function("Linus") # OUTPUT: Linus Refsnes
By default, a function must be called with the correct number of arguments. Meaning that if your function expects 2 arguments, you have to call the function with 2 arguments, not more, and not less.
If you try to call the function with 1 or 3 arguments, you will get an error:
This function expects 2 arguments, but gets only 1:
Arbitrary Arguments, *args
If you do not know how many arguments will be passed into your function, add a * before the parameter name in the function definition. This way the function will receive a tuple of arguments and can access the items accordingly:
def my_function(*kids):
print("The youngest child is " + kids[2])
my_function("Emil", "Tobias", "Linus")
Keyword Arguments
You can also send arguments with the key = value syntax. This way the order of the arguments does not matter.
def my_function(child3, child2, child1):
print("The youngest child is " + child3)
my_function(child1 = "Emil", child2 = "Tobias", child3 = "Linus")
Arbitrary Keyword Arguments, **kwargs
If you do not know how many keyword arguments will be passed into your function, add two asterisk: ** before the parameter name in the function definition.
This way the function will receive a dictionary of arguments and can access the items accordingly. This way the order of the arguments does not matter.
def my_function(**kid):
print("His last name is " + kid["lname"])
my_function(fname = "Tobias", lname = "Refsnes")
Default Parameter Value
The following example shows how to use a default parameter value. If we call the function without argument, it uses the default value:
def my_function(country = "Norway"):
print("I am from " + country)
my_function("Sweden") # Overrides "country" parameter
my_function("India")
my_function() # Uses the default value
my_function("Brazil")
Return Values
To let a function return a value, use the return statement:
def my_function(x):
return 5 * x
print(my_function(3))
print(my_function(5))
print(my_function(9))
The pass Statement
Function definitions cannot be empty, but if you for some reason have a function definition with no content, put it in the pass statement to avoid getting an error.
def myfunction():
pass
Recursion
Python also accepts function recursion, which means a defined function can call itself.
Recursion is a common mathematical and programming concept. It means that a function calls itself**. This has the benefit of meaning that you can loop through data to reach a result.**
The developer should be very careful with recursion as it can be quite easy to slip into writing a function that never terminates, or one that uses excess amounts of memory or processor power. However, when written correctly recursion can be a very efficient and mathematically elegant approach to programming.
def tri_recursion(k):
if(k > 0):
result = k + tri_recursion(k - 1) # Recursion
print(result)
else:
result = 0
return result
print("\n\nRecursion Example Results")
tri_recursion(6) # Call the function
File Handling
File handling refers to reading or writing data from files. Python provides some functions that allow us to manipulate data in the files.
open()
function
var_name = open("file name", " mode")
modes-
r
- to read the content from the file.w
- to write the content into a file.a
- to append the existing content to the file.r+
: To read and write data into the file. The previous data in the file will be overridden.w+
: To write and read data. It will override existing data.a+
: To append and read data from the file. It won’t override existing data.
close()
function
var_name.close()
read()
function
The read functions contain different methods, read()
, readline()
and readlines()
read() # return one big string
readlines() # returns a list
readline() # returns one line at a time
write()
function
This function writes a sequence of strings to the file.
write() # Used to write a fixed sequence of characters to a file
writelines() # It is used to write a list of strings
Exception Handling
An exception is an unusual condition that results in an interruption in the flow of a program.
1. try and except
A basic try-catch block in Python. When the try
block throws an error, the control goes to the except
block.
2. else
else
a block is executed if the try
block has not raised any exceptions and the code had been running successfully.
3. finally
Finally, the block will be executed even if the try block of code has been running successfully or the except
block of code is been executed. finally
, a block of code will be executed compulsorily.
try:
[Statement body block]
raise Exception()
except Exceptionname:
[Error processing block]
else:
# statements
finally:
# statement
Object Oriented Programming (OOPS)
It is a programming approach that primarily focuses on using objects and classes. The objects can be any real-world entities.
1. class
The syntax for writing a class in Python.
class class_name:
pass #statements
2. Creating an object
Instantiating an object can be done as follows:
<object-name> = <class-name>(<arguments>)
3. self parameter
The self-parameter is the first parameter of any function present in the class. It can be of a different name but this parameter is a must while defining any function into class as it is used to access other data members of the class.
4. class with a constructor
Constructor is the special function of the class which is used to initialize the objects. The syntax for writing a class with the constructor in Python.
class MyClass:
# Default constructor
def __init__(self):
self.name = "MyClass"
# A method for printing data members
def print_me(self):
print(self.name)
5. Inheritance in Python
By using inheritance, we can create a class that uses all the properties and behaviour of another class. The new class is known as a derived class or child class, and the one whose properties are acquired is known as a base class or parent class.
It provides the re-usability of the code.
class Base_class:
pass
class derived_class(Base_class):
pass
6. Types of inheritance-
- Single inheritance
- Multiple inheritance
- Multilevel inheritance
- Hierarchical inheritance
7. filter
function
The filter function allows you to process an iterable and extract those items that satisfy a given condition.
filter(function, iterable)
8. issubclass
function
Used to find whether a class is a subclass of a given class or not as follows:
issubclass(obj, classinfo) # returns true if obj is a subclass of classinfo
Iterators and Generators
Here are some of the advanced topics of the Python programming language like iterators and generators
1. Iterator
Used to create an iterator over an iterable
iter_list = iter(['Rancho', 'Raju', 'Farhan'])
print(next(iter_list))
print(next(iter_list))
print(next(iter_list))
2. Generator
Used to generate values on the fly
# A simple generator function
def my_gen():
n = 1
print('This is printed first')
# Generator function contains yield statements
yield n
n += 1
print('This is printed second')
yield n
n += 1
print('This is printed at last')
yield n
Decorators
Decorators are used to modify the behaviour of a function or a class. They are usually called before the definition of a function you want to decorate.
1. property
Decorator (getter)
@property
def name(self):
return self.__name
2. setter
Decorator
It is used to set the property 'name'
@name.setter
def name(self, value):
self.__name=value
3. deleter
Decorator
It is used to delete the property 'name'
@name.deleter #property-name.deleter decorator
def name(self, value):
print('Deleting..')
del self.__name